This program concatenates strings, for example if the first string is "c " and second string is "program" then on concatenating these two strings we get the string "c program". To concatenate two strings we use strcat function of string.h, to concatenate without using library function see another code below which uses pointers.
C programming code
#include <stdio.h>
#include <string.h>
int main()
{
char a[1000], b[1000];
printf("Enter the first string\n");
gets(a);
printf("Enter the second string\n");
gets(b);
strcat(a,b);
printf("String obtained on concatenation is %s\n",a);
return 0;
}
Output of program:
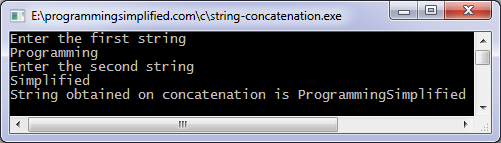
Concatenate strings without strcat function
C program to concatenate strings without using library function strcat of string.h header file. We create our own function.
#include <stdio.h>
void concatenate(char [], char []);
int main()
{
char p[100], q[100];
printf("Input a string\n");
gets(p);
printf("Input a string to concatenate\n");
gets(q);
concatenate(p, q);
printf("String obtained on concatenation is \"%s\"\n", p);
return 0;
}
void concatenate(char p[], char q[]) {
int c, d;
c = 0;
while (p[c] != '\0') {
c++;
}
d = 0;
while (q[d] != '\0') {
p[c] = q[d];
d++;
c++;
}
p[c] = '\0';
}
The first for loop in concatenate function is calculating string length so you can also use strlen function if you wish.
String concatenation using pointers
#include <stdio.h>
void concatenate_string(char*, char*);
int main()
{
char original[100], add[100];
printf("Enter source string\n");
gets(original);
printf("Enter string to concatenate\n");
gets(add);
concatenate_string(original, add);
printf("String after concatenation is \"%s\"\n", original);
return 0;
}
void concatenate_string(char *original, char *add)
{
while(*original)
original++;
while(*add)
{
*original = *add;
add++;
original++;
}
*original = '\0';
}
No comments:
Post a Comment